WordPress custom pagination for Bootstrap
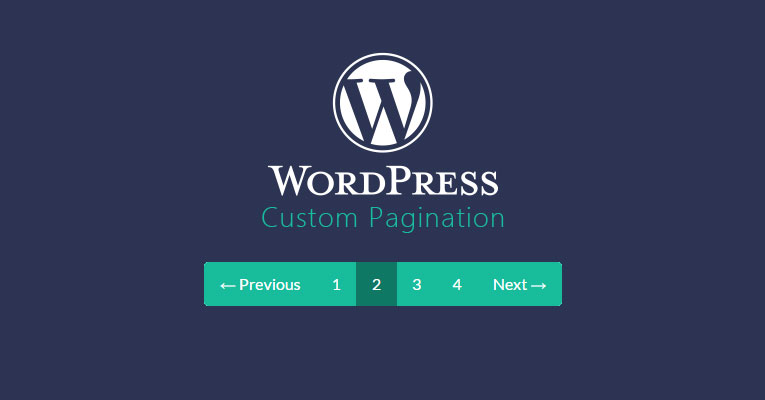
Creating custom pagination in WordPress can be a pain and a time consuming task especially if you are creating a Bootstrap based theme. WordPress echoes pagination as <a> tags while Boostrap styled pagers and pagination use <ul> and <li> tags with predefined CSS classes assigned to these elements.
In this tutorial we will cover how to create custom pagination, you should probably learn more about paginate_links so you can understand the following code. Just add the function to your functions.php file:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
function custom_pagination() { global $wp_query; $big = 999999999; $pages = paginate_links(array( 'base' => str_replace($big, '%#%', esc_url(get_pagenum_link($big))), 'format' => '?page=%#%', 'current' => max(1, get_query_var('paged')), 'total' => $wp_query->max_num_pages, 'prev_next' => false, 'type' => 'array', 'prev_next' => TRUE, 'prev_text' => '← Previous', 'next_text' => 'Next →', )); if (is_array($pages)) { $current_page = ( get_query_var('paged') == 0 ) ? 1 : get_query_var('paged'); echo '<ul class="pagination">'; foreach ($pages as $i => $page) { if ($current_page == 1 && $i == 0) { echo "<li class='active'>$page</li>"; } else { if ($current_page != 1 && $current_page == $i) { echo "<li class='active'>$page</li>"; } else { echo "<li>$page</li>"; } } } echo '</ul>'; } } |
The current page will have the “active” class so you can style it differently <li class=”active”>.
Now, let’s call the function wherever we want to display the pagination. Make sure you’re calling it outside the loop:
1 |
<?php custom_pagination(); ?> |
I hope you find this code snippet useful.
Thanks for sharing !